L2 Sequencer Uptime Feeds
Optimistic rollup protocols move all execution off the layer 1 (L1) Ethereum chain, complete execution on a layer 2 (L2) chain, and return the results of the L2 execution back to the L1. These protocols have a sequencer that executes and rolls up the L2 transactions by batching multiple transactions into a single transaction.
If a sequencer becomes unavailable, it is impossible to access read/write APIs that consumers are using and applications on the L2 network will be down for most users without interacting directly through the L1 optimistic rollup contracts. The L2 has not stopped, but it would be unfair to continue providing service on your applications when only a few users can use them.
To help your applications identify when the sequencer is unavailable, you can use a data feed that tracks the last known status of the sequencer at a given point in time. This helps you prevent mass liquidations by providing a grace period to allow customers to react to such an event.
Available networks
You can find proxy addresses for the L2 sequencer feeds at the following addresses:
- Arbitrum:
- Arbitrum mainnet: 0xFdB631F5EE196F0ed6FAa767959853A9F217697D
- OP:
- OP mainnet: 0x371EAD81c9102C9BF4874A9075FFFf170F2Ee389
- BASE:
- BASE mainnet: 0xBCF85224fc0756B9Fa45aA7892530B47e10b6433
- Metis:
- Andromeda mainnet: 0x58218ea7422255EBE94e56b504035a784b7AA204
- Scroll:
- Scroll mainnet: 0x45c2b8C204568A03Dc7A2E32B71D67Fe97F908A9
- zkSync:
- zkSync mainnet: 0x0E6AC8B967393dcD3D36677c126976157F993940
Arbitrum
The diagram below shows how these feeds update and how a consumer retrieves the status of the Arbitrum sequencer.
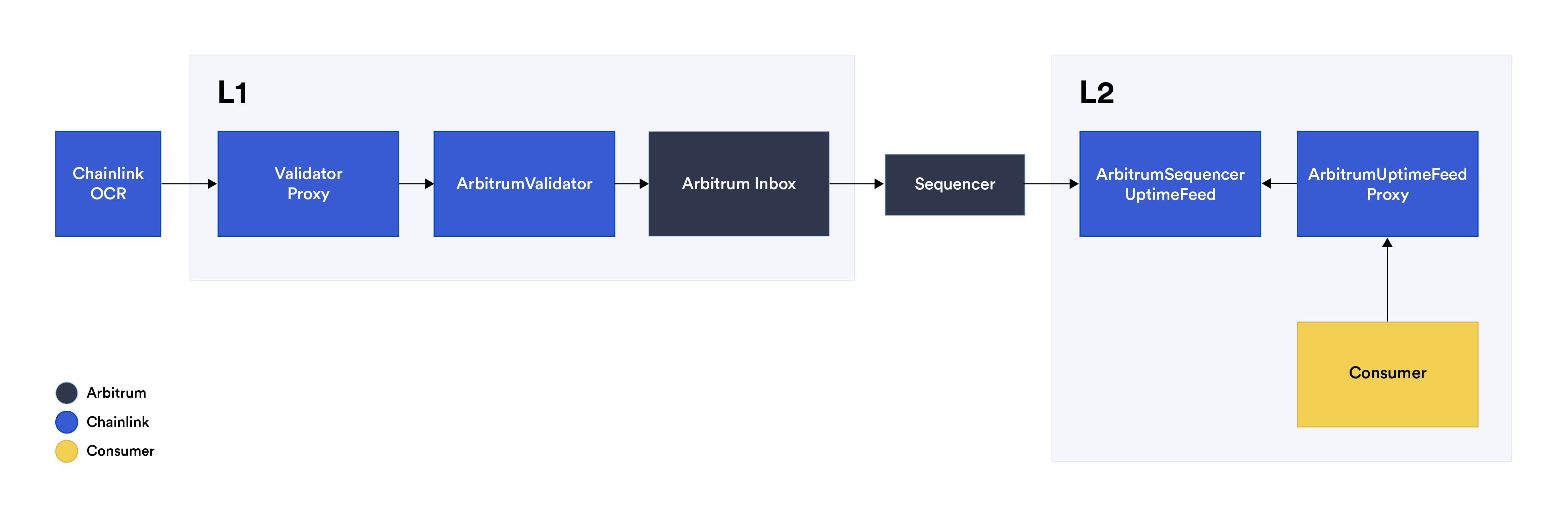
- Chainlink nodes trigger an OCR round every 30s and update the sequencer status by calling the
validate
function in theArbitrumValidator
contract by calling it through theValidatorProxy
contract. - The
ArbitrumValidator
checks to see if the latest update is different from the previous update. If it detects a difference, it places a message in the Arbitrum inbox contract. - The inbox contract sends the message to the
ArbitrumSequencerUptimeFeed
contract. The message calls theupdateStatus
function in theArbitrumSequencerUptimeFeed
contract and updates the latest sequencer status to 0 if the sequencer is up and 1 if it is down. It also records the block timestamp to indicate when the message was sent from the L1 network. - A consumer contract on the L2 network can read these values from the
ArbitrumUptimeFeedProxy
contract, which reads values from theArbitrumSequencerUptimeFeed
contract.
Handling Arbitrum outages
If the Arbitrum network becomes unavailable, the ArbitrumValidator
contract continues to send messages to the L2 network through the delayed inbox on L1. This message stays there until the sequencer is back up again. When the sequencer comes back online after downtime, it processes all transactions from the delayed inbox before it accepts new transactions. The message that signals when the sequencer is down will be processed before any new messages with transactions that require the sequencer to be operational.
OP, BASE, Metis, Scroll, and zkSync
On OP, BASE, Metis, Scroll, and zkSync, the sequencer's status is relayed from L1 to L2 where the consumer can retrieve it.
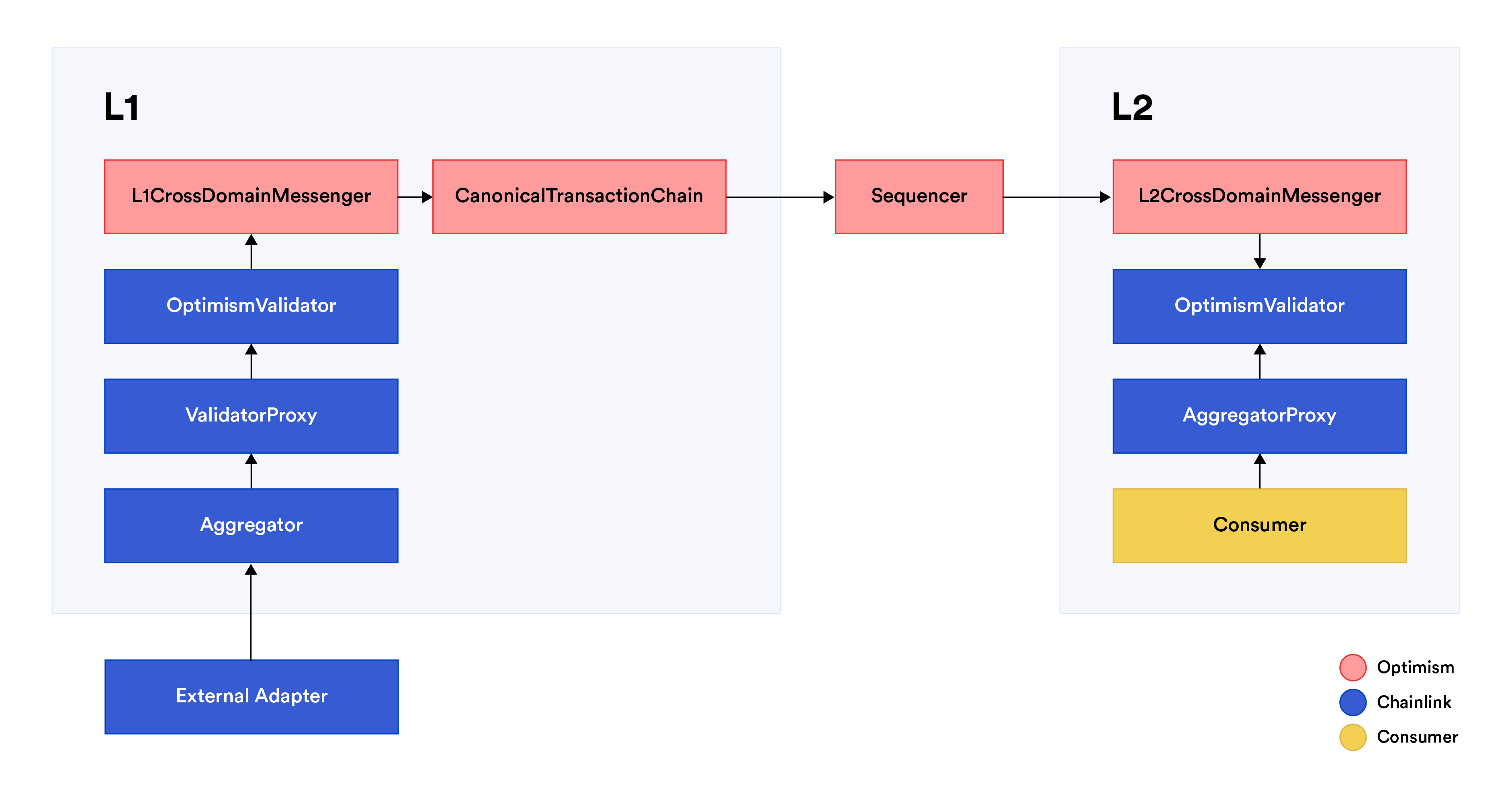
On the L1 network:
-
A network of node operators runs the external adapter to post the latest sequencer status to the
AggregatorProxy
contract and relays the status to theAggregator
contract. TheAggregator
contract calls thevalidate
function in theOptimismValidator
contract. -
The
OptimismValidator
contract calls thesendMessage
function in theL1CrossDomainMessenger
contract. This message contains instructions to call theupdateStatus(bool status, uint64 timestamp)
function in the sequencer uptime feed deployed on the L2 network. -
The
L1CrossDomainMessenger
contract calls theenqueue
function to enqueue a new message to theCanonicalTransactionChain
. -
The
Sequencer
processes the transaction enqueued in theCanonicalTransactionChain
contract to send it to the L2 contract.
On the L2 network:
-
The
Sequencer
posts the message to theL2CrossDomainMessenger
contract. -
The
L2CrossDomainMessenger
contract relays the message to theOptimismSequencerUptimeFeed
contract. -
The message relayed by the
L2CrossDomainMessenger
contains instructions to callupdateStatus
in theOptimismSequencerUptimeFeed
contract. -
Consumers can then read from the
AggregatorProxy
contract, which fetches the latest round data from theOptimismSequencerUptimeFeed
contract.
Handling outages on OP, BASE, Metis, Scroll, and zkSync
If the sequencer is down, messages cannot be transmitted from L1 to L2 and no L2 transactions are executed. Instead, messages are enqueued in the CanonicalTransactionChain
on L1 and only processed in the order they arrived later when the sequencer comes back up. As long as the message from the validator on L1 is already enqueued in the CTC
, the flag on the sequencer uptime feed on L2 will be guaranteed to be flipped prior to any subsequent transactions. The transaction that flips the flag on the uptime feed will be executed before transactions that were enqueued after it. This is further explained in the diagrams below.
When the Sequencer is down, all L2 transactions sent from the L1 network wait in the pending queue.
- Transaction 3 contains Chainlinkās transaction to set the status of the sequencer as being down on L2.
- Transaction 4 is a transaction made by a consumer that is dependent on the sequencer status.
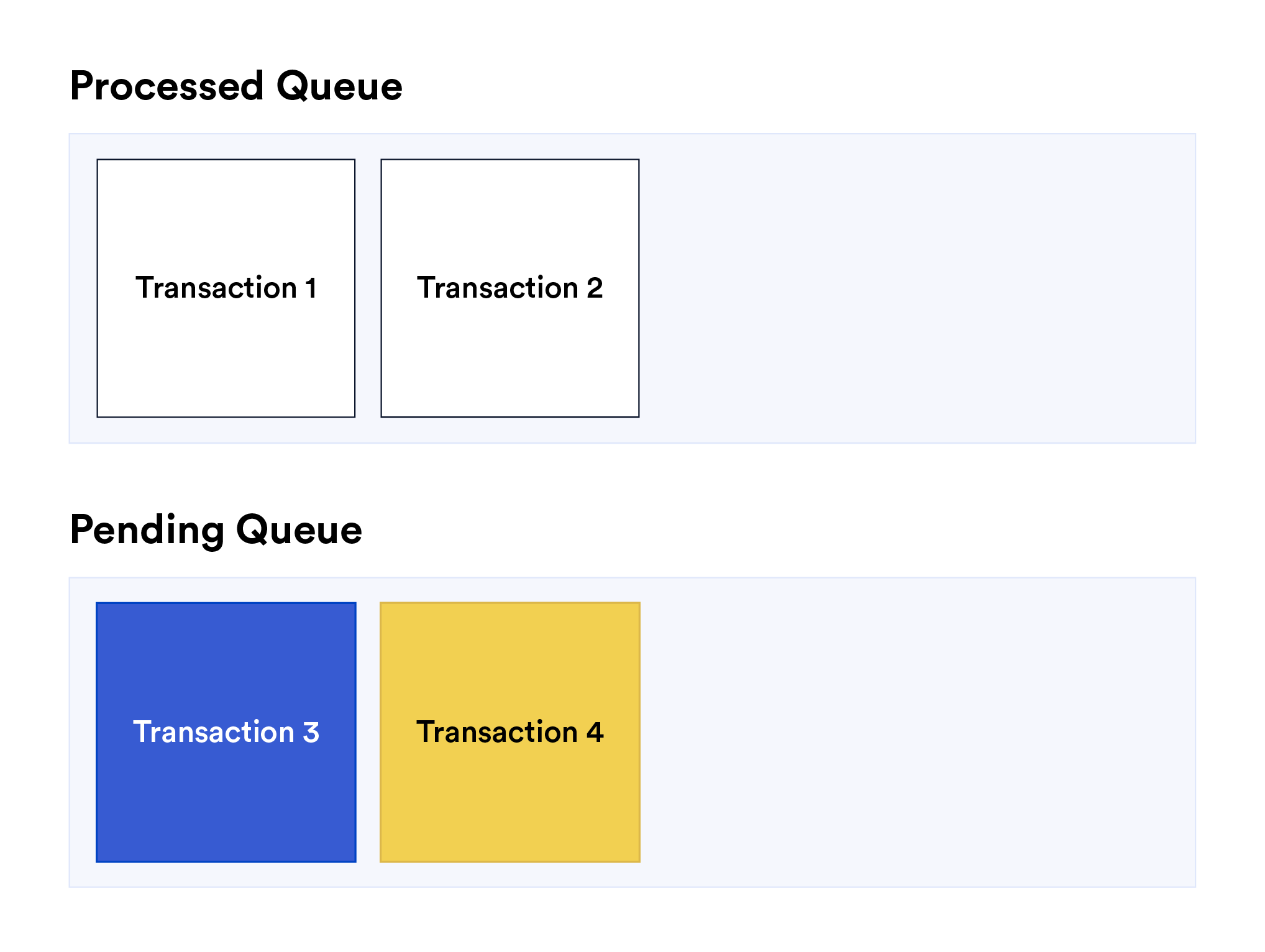
After the sequencer comes back up, it moves all transactions in the pending queue to the processed queue.
- Transactions are processed in the order they arrived so Transaction 3 is processed before Transaction 4.
- Because Transaction 3 happens before Transaction 4, Transaction 4 will read the status of the Sequencer as being down and responds accordingly.
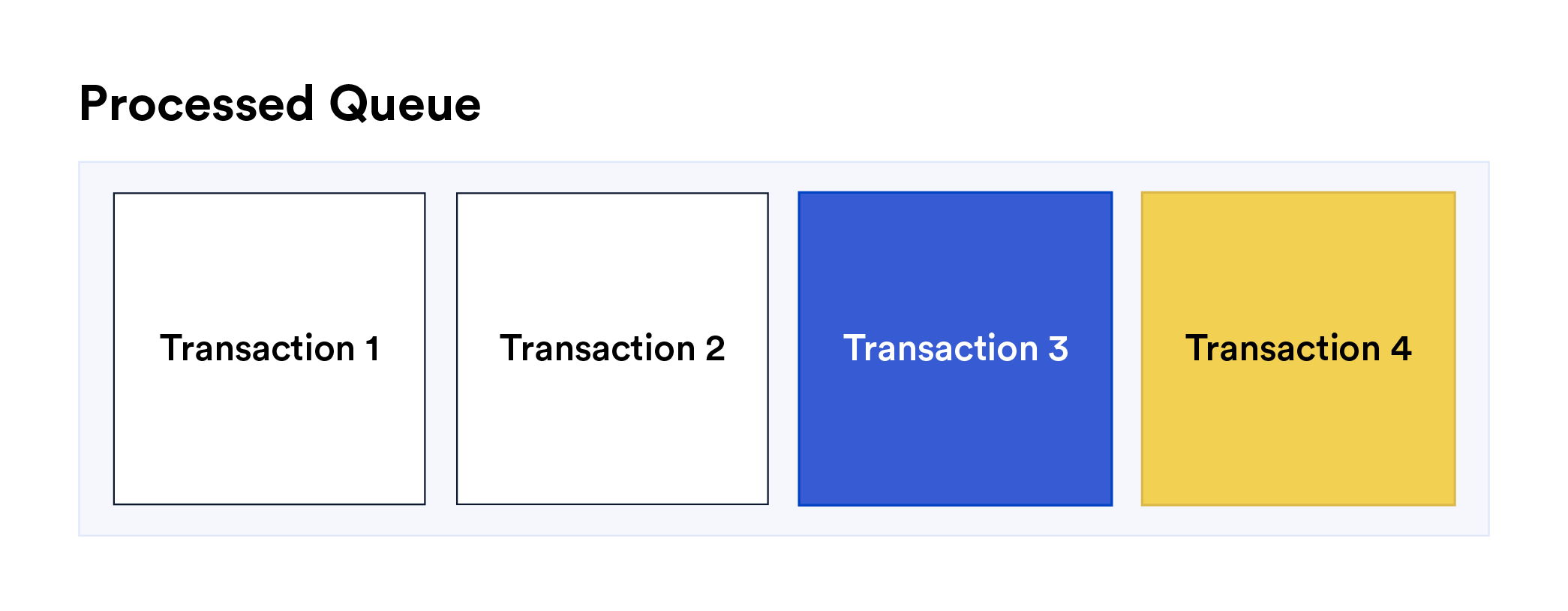
Example code
This example code works on the Arbitrum, OP, and Metis networks. Create the consumer contract for sequencer uptime feeds similarly to the contracts that you use for other Chainlink Data Feeds. Configure the constructor using the following variables:
- Configure the
sequencerUptimeFeed
object with the sequencer uptime feed proxy address for your L2 network. - Configure the
dataFeed
object with one of the Data Feed proxy addresses that are available for your network.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.7;
import {AggregatorV2V3Interface} from "@chainlink/contracts/src/v0.8/shared/interfaces/AggregatorV2V3Interface.sol";
/**
* THIS IS AN EXAMPLE CONTRACT THAT USES HARDCODED VALUES FOR CLARITY.
* THIS IS AN EXAMPLE CONTRACT THAT USES UN-AUDITED CODE.
* DO NOT USE THIS CODE IN PRODUCTION.
*/
contract DataConsumerWithSequencerCheck {
AggregatorV2V3Interface internal dataFeed;
AggregatorV2V3Interface internal sequencerUptimeFeed;
uint256 private constant GRACE_PERIOD_TIME = 3600;
error SequencerDown();
error GracePeriodNotOver();
/**
* Network: OP Mainnet
* Data Feed: BTC/USD
* Data Feed address: 0xD702DD976Fb76Fffc2D3963D037dfDae5b04E593
* Uptime Feed address: 0x371EAD81c9102C9BF4874A9075FFFf170F2Ee389
* For a list of available Sequencer Uptime Feed proxy addresses, see:
* https://docs.chain.link/docs/data-feeds/l2-sequencer-feeds
*/
constructor() {
dataFeed = AggregatorV2V3Interface(
0xD702DD976Fb76Fffc2D3963D037dfDae5b04E593
);
sequencerUptimeFeed = AggregatorV2V3Interface(
0x371EAD81c9102C9BF4874A9075FFFf170F2Ee389
);
}
// Check the sequencer status and return the latest data
function getChainlinkDataFeedLatestAnswer() public view returns (int) {
// prettier-ignore
(
/*uint80 roundID*/,
int256 answer,
uint256 startedAt,
/*uint256 updatedAt*/,
/*uint80 answeredInRound*/
) = sequencerUptimeFeed.latestRoundData();
// Answer == 0: Sequencer is up
// Answer == 1: Sequencer is down
bool isSequencerUp = answer == 0;
if (!isSequencerUp) {
revert SequencerDown();
}
// Make sure the grace period has passed after the
// sequencer is back up.
uint256 timeSinceUp = block.timestamp - startedAt;
if (timeSinceUp <= GRACE_PERIOD_TIME) {
revert GracePeriodNotOver();
}
// prettier-ignore
(
/*uint80 roundID*/,
int data,
/*uint startedAt*/,
/*uint timeStamp*/,
/*uint80 answeredInRound*/
) = dataFeed.latestRoundData();
return data;
}
}
The sequencerUptimeFeed
object returns the following values:
answer
: A variable with a value of either0
or1
- 0: The sequencer is up
- 1: The sequencer is down
startedAt
: This timestamp indicates when the sequencer feed changed status. When the sequencer comes back up after an outage, wait for theGRACE_PERIOD_TIME
to pass before accepting answers from the data feed. SubtractstartedAt
fromblock.timestamp
and revert the request if the result is less than theGRACE_PERIOD_TIME
.- The
startedAt
variable returns0
only on Arbitrum when the Sequencer Uptime contract is not yet initialized. For L2 chains other than Arbitrum,startedAt
is set toblock.timestamp
on construction andstartedAt
is never0
. After the feed begins rounds, thestartedAt
timestamp will always indicate when the sequencer feed last changed status.
- The
If the sequencer is up and the GRACE_PERIOD_TIME
has passed, the function retrieves the latest answer from the data feed using the dataFeed
object.